Introduction
In this post, we will build a Long Straddle Options Trading strategy on TradingView. We will automate this trading strategy using AutoTrader Web. AutoTrader Web currently supports Supports leading Indian Stock Brokers.
The strategy is for learning & demonstration purposes only. We DO NOT promise any returns.
Admin
Demo
Strategy Logic
A long straddle is an options strategy that involves purchasing both a long call and a long put on the same underlying asset with the same expiration date and strike price.
This strategy will be beneficial when the underlying asset makes a big move in any direction. If the move is upwards, then a trader will benefit on his call option. If the move is downwards, then a trader will suffer on his put option.
If the market does not move a lot then a trader will suffer a maximum of the total premium he paid for purchasing call & put option.
We are using moving average indicator in this strategy. As we need a big move in the underlying asset to be profitable, a trader should consider using higher values for short & long term moving average parameters.
TradingView does not support option charts. A trader shall run this strategy on the underlying asset. This asset can be a stock or an index or their respective future contracts. On receiving a signal, we will pass AutoTrader Web’s option symbols through the webhook alert.
Exit Strategy: For now, we have assumed that the time needed for a big move in the underlying asset would be a lot. So, a trader will manually exit the positions. In future, we may add the code to automate the exit.
Pre-requisite
- AutoTrader Web account
- TradingView account
- Trading account with any of the supported brokers mentioned above
Setup
- Open an account on TradingView (if you do not have it)
- Register on AutoTrader Web
- Add your Trading Account
Process
Step 1: Create a strategy
- Go to TradingView
- Click on Chart option on the top
- Choose the stock/derivative you want to work on by selecting the Symbol (you can see the symbol on LEFT TOP section of the chart screen)
- You can select any symbol (stock, derivatives on NSE, BSE or MCX)
- Click on Pine Editor (it can be found on BOTTOM section of the chart screen)
- Change the name from “Untitled Script” to “Long Straddle“
- Remove existing content from the script and add following strategy code
- Feel free to do any modifications of your choice
- Note: We are using AutoTrader Web’s public function library. You should always use the latest version of this library, by changing the version number (line no. 7) to the most recent one.
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pritesh-StocksDeveloper
//@version=5
strategy("Long Straddle", overlay=true, calc_on_every_tick = true)
import Pritesh-StocksDeveloper/StocksDeveloper_AutoTraderWeb/6 as autotrader
// A long straddle is profitable when a market is expected to make large
// moves in any direction. It involves buying call & put at the same strike,
// so it is essentially a "market or direction neutral" strategy.
i_account = input.string(defval = "ACC_NAME", title = "Pseudo/Group Account",
tooltip = "Your Pseudo or Group account name", confirm = true,
group = "Account")
i_group = input.bool(defval = false, title = "Group Account",
tooltip = "Set it to true if you are using a Group account",
confirm = true, group = "Account")
i_exchange = input.string(defval = "NSE", title = "Exchange of Symbol",
tooltip = "Exchange (Ex. NSE, BSE, MCX)", confirm=true,
group = "Instrument")
i_symbol = input.string(defval = "BANKNIFTY", title = "Underlier Symbol",
tooltip = "Underlier symbol (Ex. NIFTY, CRUDEOIL, USDINR)", confirm=true,
group = "Instrument")
i_expiry = input.string(defval = "27-JAN-2022", title="Expiry (DD-MMM-YYYY)",
tooltip = "Expiry date for the option contracts", confirm = true,
group = "Instrument")
// Short and Long moving avg. period input parameters
i_shortPeriod = input.int(title = "Short MA Period", defval = 50, minval = 2,
maxval = 50, confirm = true, group = "Strategy")
i_longPeriod = input.int(title = "Long MA Period", defval = 200, minval = 3,
maxval = 200, confirm = true, group = "Strategy")
i_quantity = input.int(title = "Quantity", defval = 25, minval = 1,
tooltip = "Quantity (in multiples of lot size)",
confirm = true, group = "Strategy")
i_productType = input.string(defval = "NORMAL", title="Product Type",
tooltip = "Product Type (INTRADAY, DELIVERY, NORMAL)", confirm = true,
group = "Strategy")
// Gap between option strikes (Example: BANKNIFTY option strikes has a gap
// of 100 points)
i_gap = input.int(defval = 100, title = "Gap between option strikes",
tooltip = "Example: BANKNIFTY option strikes have a gap of 100",
confirm = true, group = "Strategy")
// Some brokers do not allow MARKET orders in options, so we use LIMIT orders
i_use_limit_ot = input.bool(defval = false, title = "Use LIMIT Order",
tooltip = "Set it to true if your broker has blocked MARKET orders. You must set limit order price if this is set to true.",
confirm = true, group = "Strategy")
i_price = input.float(title = "Limit Order Price", defval = 100, minval = 1,
tooltip = "Limit order price (set it a lot higher than option LTP, so that your order will execute just like a MARKET order)",
confirm = true, group = "Strategy")
// A function to prepare long straddle alert message
prepareLongStraddle(string exchange, string symbol, string expiry, int gap,
string account, bool group, int quantity, string productType,
bool useLimitOrder, float price) =>
// Calculate the strike which is closest to current live price
strike = autotrader.calcClosestStrike(close, gap)
// Prepare call option symbol as per AutoTrader Web's format
callSymbol = autotrader.prepareOptionSymbol(underlier = symbol,
expiry = expiry, optionType = "CE", strike = strike)
// Prepare put option symbol as per AutoTrader Web's format
putSymbol = autotrader.prepareOptionSymbol(underlier = symbol,
expiry = expiry, optionType = "PE", strike = strike)
// Select order type based on user input
orderType = (useLimitOrder) ? "LIMIT" : "MARKET"
// Use price based on order type
prc = (useLimitOrder) ? price : 0
// Prepare alert message for placing two orders (call buy & put buy)
autotrader.preparePlaceOrderAlertMessageForTwoOrders(account = account,
symbol = callSymbol, tradeType = "BUY", group = group,
exchange = exchange, quantity = quantity, productType = productType,
orderType = orderType, price = prc,
account2 = account, symbol2 = putSymbol, tradeType2 = "BUY",
group2 = group, exchange2 = exchange, quantity2 = quantity,
productType2 = productType, orderType2 = orderType, price2 = prc,
comments = "Long Straddle")
// Calculate moving averages
shortAvg = ta.sma(close, i_shortPeriod)
longAvg = ta.sma(close, i_longPeriod)
// Plot moving averages
plot(series = shortAvg, color = color.red, title = "Short MA", linewidth = 2)
plot(series = longAvg, color = color.blue, title = "Long MA", linewidth = 2)
// Long/short condition
longCondition = ta.crossover(shortAvg, longAvg)
shortCondition = ta.crossunder(shortAvg, longAvg)
// If either long or short condition is true then message will be set to
// alert json, otherwise it will be blank
isLongOrShort = longCondition or shortCondition
message = (isLongOrShort) ? prepareLongStraddle(i_exchange, i_symbol,
i_expiry, i_gap, i_account, i_group, i_quantity, i_productType,
i_use_limit_ot, i_price) : ""
// When we expect a large movement in the underlier, then enter into a
// long straddle
// Note that the strategy.long type is meaningless for us because
// we are entering into a long strddle.
// A long straddle buys call & put at the same strike,
// so it is essentially a market or direction neutral strategy.
strategy.entry(id = "Long", direction = strategy.long, when = longCondition,
alert_message = message)
strategy.entry(id = "Short", direction = strategy.short, when = shortCondition,
alert_message = message)
Step 2: Add strategy to chart
- Make sure you selected the symbol of the underlying asset or it’s future (Ex. BANKNIFTY)
- Click Add to Chart button (see image below)
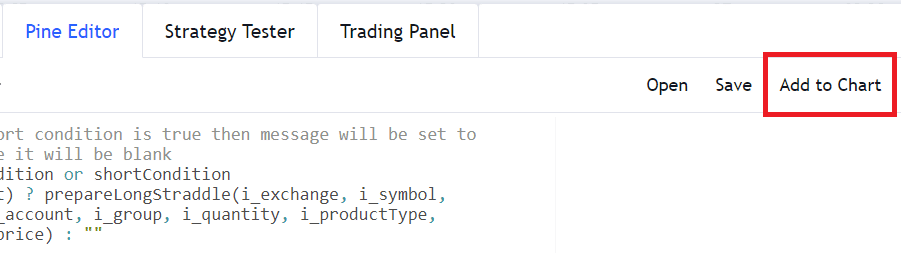
Step 3: Set strategy parameters
Parameters have been grouped based on their use-case.
- ACCOUNT
- Pseudo/Group Account – The name of the Pseudo or Group account that you want to trade into
- To trade into a single account, you will most likely use a Pseudo account
- To trade into one or more accounts, you shall use a Group account
- Group Account – Check this box if you are using a Group account
- Pseudo/Group Account – The name of the Pseudo or Group account that you want to trade into
- INSTRUMENT
- We use AutoTrader Web’s broker independent instruments. You can use this instrument search tool.
- Exchange of Symbol – Exchange of the symbol (Ex. NSE, BSE, MCX)
- Underlier Symbol – Symbol of the underlying asset (Ex. BANKNIFTY). The strategy uses this parameter to build option symbol
- Expiry – The expiry of the options you want to trade in DD-MMM-YYYY format
- STRATEGY
- Short MA Period – Short term moving average value
- Long MA Period – Long term moving average value
- Quantity – Quantity (should be multiple of lot size) (Ex. to buy 1 lot of BANKNIFTY use quantity as 25)
- Product Type – Use NORMAL (for carryforward positions) or INTRADAY (for intraday positions)
- Gap between Option Strikes – The difference between two consecutive option strikes (Example: BANKNIFTY option strikes has a gap of 100 points)
- Use LIMIT Order – Tick this if your broker does not allow MARKET orders in options
- Limit Order Price – Applicable only if you are using LIMIT orders. The value should be a lot higher than the current LTP but should be below circuit limit. This will make sure that the options buy order will execute just like a MARKET order. (Example: If banknifty is trading close to 35000 and both call & put of 35000 strike are trading at say around 700/- then you should enter this price at a higher value. So something like 1200/-)
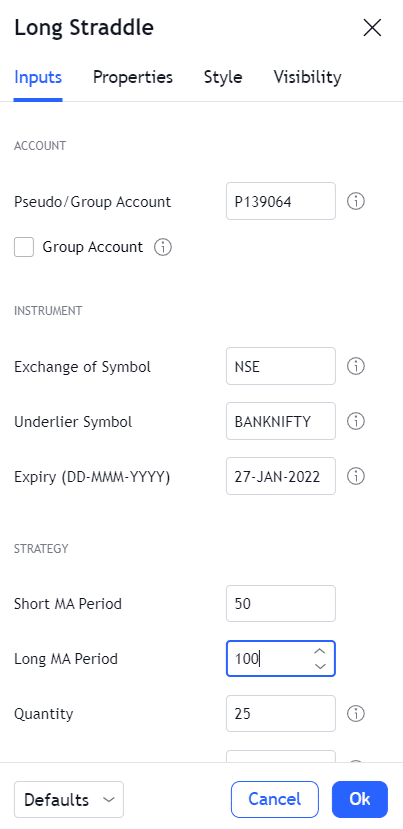
Step 4: Create alert
- Press (Alt + A) to bring up create alert window. Alternatively you can also use the buttons as shown in below screenshot.
- Condition – Long Straddle
- Alert Actions
- Webhook – Use the following URL. Remember you need to add your API key. You can find you API key from AutoTrader Web menu (Settings – API Key)
- https://tv.stocksdeveloper.in/?apiKey=XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
- You can use any other alert actions as per your own preference
- Webhook – Use the following URL. Remember you need to add your API key. You can find you API key from AutoTrader Web menu (Settings – API Key)
- Alert Name – Any meaningful name (Ex. Long Straddle Alert)
- Message – We are passing the content of the alert from the strategy. Hence, we need to use the following placeholder (See screenshot below).
- {{strategy.order.alert_message}}

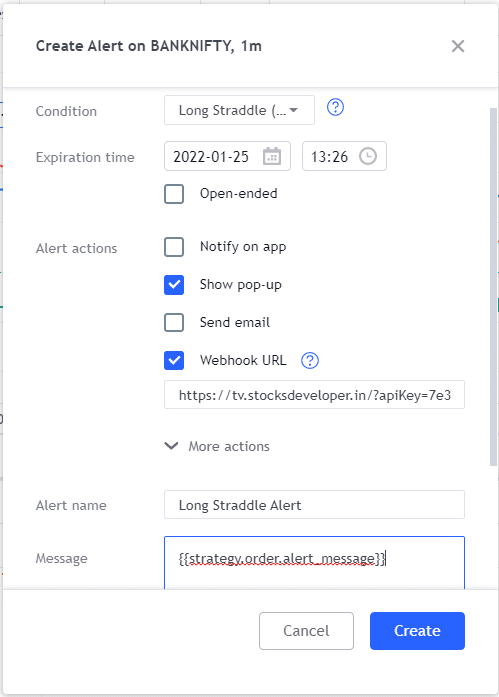
Disclaimer
We have provided this strategy for demonstration purposes. Users can use this as learning or reference material. We do not promise any kind of financial returns based on this strategy.
Ideally, users should research and build their own strategies & backtest before making them live.
Hello,
Just want some info.
Does Trading view support banknifty and nifty options chart with strangle and straddle strategy charts?
January 26, 2022 at 5:34 pmAs seen in the demo, you can trade in options but your strategy will run on the underlier index or it’s future. This is because as far as we know, TV does not support option charts.
January 28, 2022 at 3:42 pmI am looking for long straddle algo program for angel api
May 23, 2022 at 2:45 amThe strategy given here works on all supported brokers including Angel.
May 25, 2022 at 11:14 pmSir , I would like close the previous open option position before taking new option position, Pl. help me in this regard , how can i prepare an message to auto square off previous position in options.Thank U sir.
June 24, 2022 at 4:16 amI want to use another strategy where I buy only one of the options: either CE or PE. Is there any demo for this? What shall I use in place of autotrader.preparePlaceOrderAlertMessageForTwoOrders given in the current demo
April 21, 2023 at 4:11 pm