This problem is mostly faced by traders who are new to AmiBroker programming. They copy code to place orders from sample afl into their own afl. The code looks like below:
if ( LastValue(Buy) == True )
{
placeOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "MARKET", AT_PRODUCT_TYPE,
AT_QUANTITY, buyPrice, defaultTriggerPrice(), True);
}
The problem is that the IF condition written before placeOrder functions might not match with your afl’s buy/sell condition. If you remove if block & just keep the placeOrder function then you will see order being fired every time your strategy runs.
Solution
First and foremost, you need to understand the responsibility:
- If the placeOrder() function is being invoked from your code, then it is our responsibility
- In this case, the problem is with AutoTrader
- If the placeOrder() function is NOT being invoked from your code, then it is your responsibility
- In this case, the problem is with your strategy code
How to find whether placeOrder*() is being invoked?
If you are new to AmiBroker, please refer to AmiBroker Strategy Debugging. You need to understand that we are API providers, we provide functions & you as a developer need to use them at appropriate places. So the solution is to make sure that the condition inside if block matches your buy/sell condition. You can take developer’s help if needed. This is a development related issue (caused by lack of afl programming knowledge) & it cannot be covered by support. You can also use _TRACE function to print log messages and understand the code execution workflow. See below:
_TRACE("Outside Place Order IF Block");
if ( LastValue(Buy) == True )
{
_TRACE("Inside Place Order BUY IF Block");
placeOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "MARKET", AT_PRODUCT_TYPE,
AT_QUANTITY, buyPrice, defaultTriggerPrice(), True);
}
if ( LastValue(Sell) == True )
{
_TRACE("Inside Place Order SELL IF Block");
placeOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"SELL", "MARKET", AT_PRODUCT_TYPE,
AT_QUANTITY, sellPrice, defaultTriggerPrice(), True);
}
Now check AmiBroker log window (Menu: Window -> Log).
- Enable both Internal & External Trace Output
- Check all 3 tabs highlighted below for any signs of error
Use bar replay feature or AmiBroker or run your strategy on live data. Make sure it is being run over a price movement which should generate an order as per your logic (Example, run it over an area where signal is generated.)
If you see the message from _TRACE statement here, then the problem is with AutoTrader. Please report it to our support team. If you do not see anything, then the problem is with your strategy code.
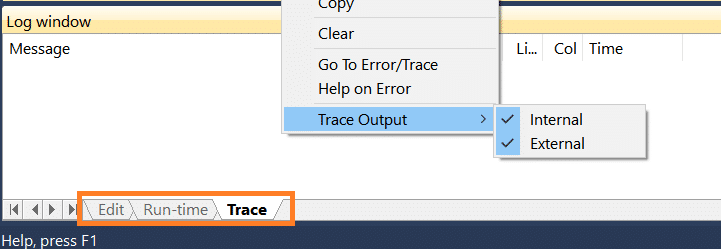