MetaTrader users should use the library that we provide in order to achieve automation. In this section, we will look at how it is done.
The library is fully compatible with MT4 & MT5. In case you face any problems, try manual installation as per steps given below or contact the support team for help.
Migration
Those migrating from our previous generation AutoTrader system should take a note that the API functions have changed. Most notable change is the addition of Pseudo Account which is an additional parameter in all the functions.
Installation
- Install AutoTrader Desktop client
- Start Desktop client
- Go to the Settings tab
- Click MetaTrader Library Install button
- The system will automatically try to locate MetaTrader folders and do the installation
Manual Installation
Just in case, the installation fails; you can try doing a manual installation.
- Download library
- Open it with WinZip or WinRar & extract the files
- Copy the files from Include folder to your MetaTrader’s Include folder.
- A sample path is given below, you need to find appropriate path on your computer
- C:\Users\<windows_user>\AppData\Roaming\MetaQuotes\Terminal\F762D69EEEA9B4430D7F17C82167C844\MQL5\Include
- If you are using MT4, then the folder above will be MQL4
Client Setup
- Install & open AutoTrader Desktop client
- Go to it’s settings tab & change the communication path as given below. Make sure you find similar path on your computer
- C:\Users\<windows_user>\AppData\Roaming\MetaQuotes\Terminal\Common\Files\autotrader
- Note: The desktop client must be running & in MONITORING state for the APIs to work.
Integration
Once the library is installed, then next step is to integrate it into your trading strategy.
Code
As with any other library, in order to use it’s functions in your strategy code; you must include it in your strategy’s code at the top.
#include <autotrader.mqh>
That’s about it. Now you are all set to use the API functions provided in the library.
Regular Order
string id = placeOrder(AT_ACCOUNT,
NSE, "SBIN", SELL, LIMIT, INTRADAY, 1,
192.44, 0.0, true);
Bracket Order
string id = placeBracketOrder(AT_ACCOUNT,
NSE, "SBIN", BUY, LIMIT, 1,
192, 0.0, 1, 1, 0, true);
Cover Order
string id = placeCoverOrder(AT_ACCOUNT,
NSE, "SBIN", BUY, LIMIT, 1,
192, 190.5, true);
Cancel Order
string orderId = placeOrder(AT_ACCOUNT,
NSE, "SBIN", SELL, LIMIT, INTRADAY, 1,
192.44, 0.0, true);
// You can even store the orderId
// in a static variable
// Somewhere later in your code,
// when you want to cancel this order
cancelOrder(AT_ACCOUNT, orderId);
API Functions
All API functions are available inside “Include\autotrader.mqh“. Do NOT modify this file.
Note: A detailed explanation is provided for each function along with examples in the API documentation.
Parameters
The library defines certain parameters that you can set in the chart parameters window. They are given below:
- AT_ACCOUNT: Pseudo account
- AT_EXCHANGE: Instrument’s exchange
- AT_SYMBOL: Instrument’s symbol
- AT_PRODUCT_TYPE: Default Product type
- AT_QUANTITY: Default Quantity
- AT_PRICE_PRECISION: Price precision for rounding
- AT_DEBUG: Print additional logs
- AT_AVOID_REPEAT_ORDER_DELAY: Avoid repeat order delay (in seconds)
Broker independent instrument’s Exchange & Symbol can be looked up on this global instruments search tool.
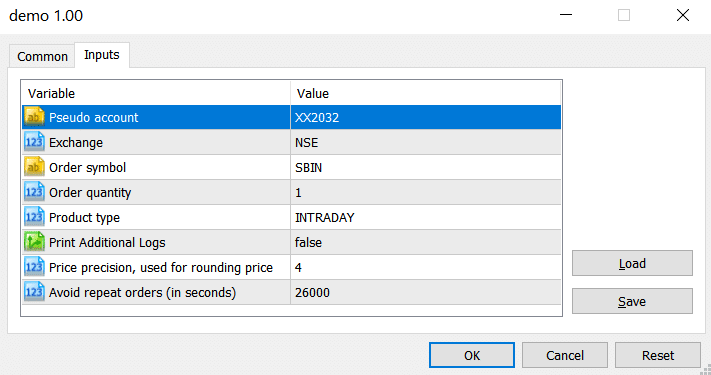
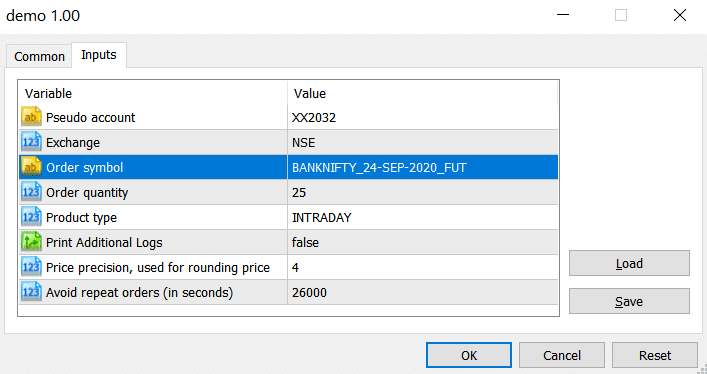
Logs
Here’s a look at sample logs that print information of the order being placed.

Trading in Multiple Accounts
There are two approaches to achieve trading into multiple accounts. You can go through them in Trading Strategies For Multiple Accounts.
Approach 1: One Strategy Instance Per Account
To trade in multiple accounts in AmiBroker, you need to run multiple charts or scanners. You need to have one chart/scanner per account.
Approach 2: One Strategy for All Accounts
All the API functions provided by us take account number as a parameter. So instead of using just a single AT_ACCOUNT chart parameter, you can always hard-code the account in the code. Here is a sample:
// Place order in ACC1
placeOrder("ACC1", AT_EXCHANGE, AT_SYMBOL, BUY, MARKET,
AT_PRODUCT_TYPE, AT_QUANTITY, 0, defaultTriggerPrice(), false);
// Place order in ACC2
placeOrder("ACC2", AT_EXCHANGE, AT_SYMBOL, BUY, MARKET,
AT_PRODUCT_TYPE, 0, buyPrice, defaultTriggerPrice(), false);
GitHub
If you are interested to look at the code of this library, it is available on GitHub.