AmiBroker users should use the library that we provide in order to achieve automation. In this section, we will look at how it is done.
Demos
All Amibroker demos are available in this YouTube playlist.
Installation
- Install AutoTrader Desktop client
- Start Desktop client
- Go to the Settings tab
- Click Amibroker Library Install button
- Select the directory where your Amibroker is installed
- Default path: C:\Program Files\AmiBroker
- Once you select the directory, click “Install Here“
- Wait for about a minute to allow the installation to complete
- You will see a message “Installation completed successfully“
The installation step downloads AFL library files and copies them to “Formulas\Include” folder in your Amibroker installation.
Just make sure the IPC directory matches in AFL library as well as Desktop client. The only difference is that AmiBroker needs a double backslash (\\) as the separator.
- Look for following entry in “Formulas\Include\autotrader-ipc.afl“
- IPC_DIR = “C:\\Users\\<username>\\autotrader”;
- On desktop client, go to settings tab
- Make sure the IPC Path matches “Communication folder” value
Integration
Once the library is installed, then next step is to integrate it into your trading strategy. It is fairly simple and you can even look at the samples available in “Formulas\AutoTraderWeb” folder (look at Charts tab in Amibroker).
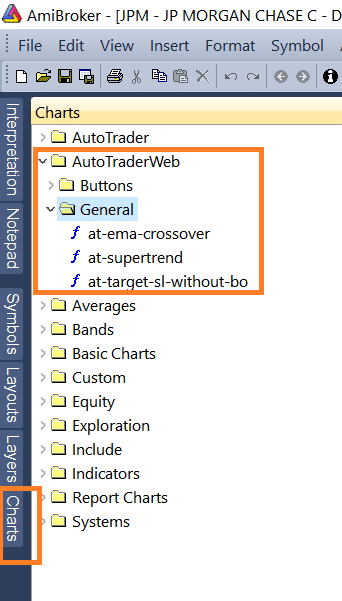
Warning: You should not modify these files as they are overwritten when you re-install the library. Use these files as a reference and copy the code into new AFL files (if you need). Make sure your personal AFL files are NOT in AutoTraderWeb folder.
Code
As with any other library, in order to use it’s functions in your strategy code; you must include it in your strategy’s afl at the top.
#include <autotrader.afl>
That’s about it. This afl internally includes all dependencies. Now you are all set to use the API functions provided in the library.
Regular Order
orderId = placeOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "MARKET", AT_PRODUCT_TYPE, AT_QUANTITY,
buyPrice, defaultTriggerPrice(), True);
Bracket Order
orderId = placeBracketOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "LIMIT", AT_QUANTITY,
buyPrice, defaultTriggerPrice(), 5, 3, 1, True);
Cover Order
stoplossTriggerPrice = buyPrice - 5;
orderId = placeCoverOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "LIMIT", AT_QUANTITY,
buyPrice, stoplossTriggerPrice, True);
Cancel Order
orderId = placeOrder(AT_ACCOUNT, AT_EXCHANGE, AT_SYMBOL,
"BUY", "MARKET", AT_PRODUCT_TYPE, AT_QUANTITY,
buyPrice, defaultTriggerPrice(), True);
// You can save this orderId in a static variable
saveStaticVariableText(AT_ACCOUNT, "ORDER_ID", orderId);
// Somewhere later in your code,
// when you want to cancel this order
// Read orderId from static variable
orderId = readStaticVariableText(AT_ACCOUNT, "ORDER_ID");
// Cancel the order
cancelOrder(AT_ACCOUNT, orderId);
API Functions
All API functions are available inside “Formulas\Include\autotrader-api.afl“. Do NOT modify this afl file.
Note: A detailed explanation is provided for each function along with examples in the API documentation.
Parameters
The afl library defines certain parameters that you can set in the chart/exploration parameters window. They are given below:
- AT_ACCOUNT: Pseudo account
- AT_EXCHANGE: Instrument’s exchange
- AT_SYMBOL: Instrument’s symbol
- AT_PRODUCT_TYPE: Default Product type
- AT_QUANTITY: Default Quantity
- AT_PRICE_PRECISION: Price precision for rounding
- AT_DEBUG: Print additional logs
- AT_AVOID_REPEAT_ORDER_DELAY: Avoid repeat order delay (in seconds)
Broker independent instrument’s Exchange & Symbol can be looked up on this global instruments search tool.
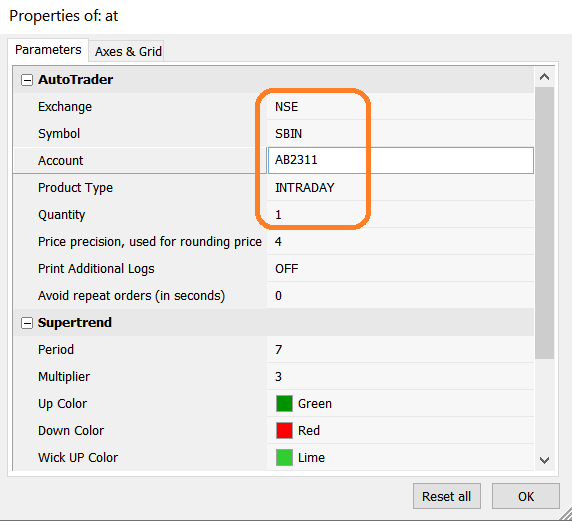
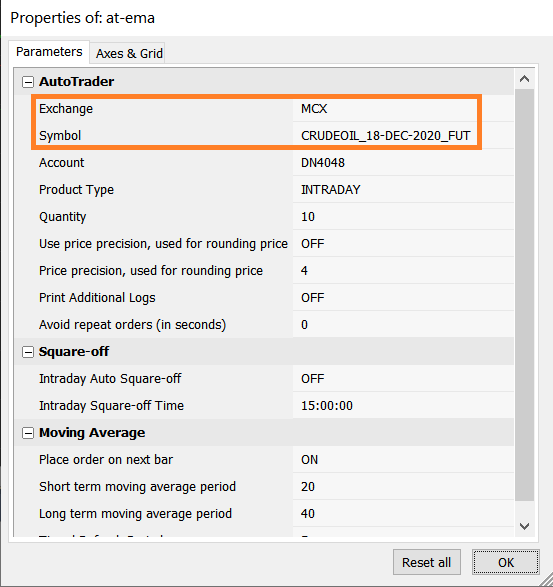
Sample Strategies
Sample strategies are available under “Formulas\AutoTraderWeb“.
Button Trading
There are many sample button trading AFLs available under “Formulas\AutoTraderWeb\Buttons“. We recommend you to read & understand the afl code before using them.
A detailed guide on button trading is available on AmiBroker Multi-Account Button Trading.
Scanner
The most important thing you should remember is the use of Name() function to get the scanner symbol. You should not use AT_SYMBOL parameter defined by our library.
You can easily map your datafeed provider’s symbol to AutoTrader Web’s symbol using csv file.
Also, you can trade different quantity per scanner symbol by mapping the quantity in a csv file.
Look for a sample AutoTraderWeb\General\at-supertrend-scanner.afl. You can enable csv files & set the file path in chart parameters as shown below.
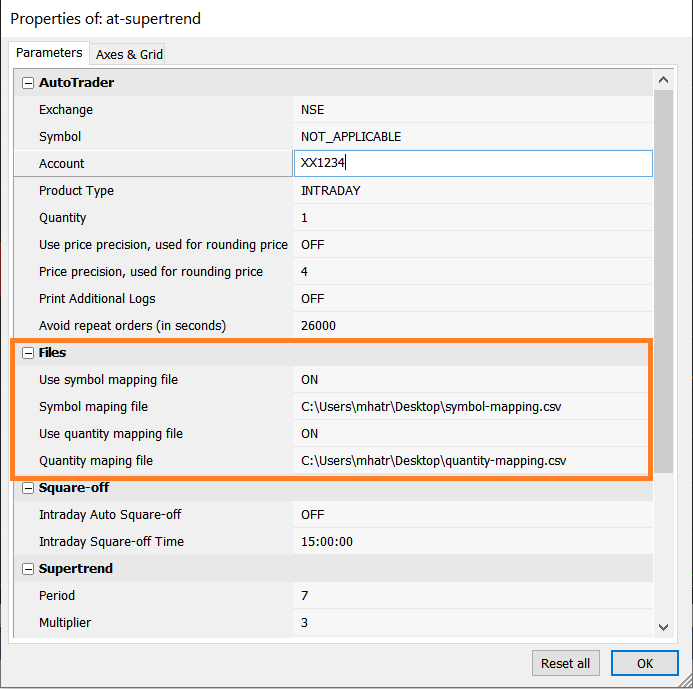
Sample “symbol-mapping.csv”
- Column 1: Datafeed provider symbol
- Column 2: AutoTrader Web’s broker independent symbol
- Give a comma (,) at the end of each line
- See sample entries below, copy them & paste it into a csv file
BANKNIFTY-I,BANKNIFTY_24-SEP-2020_FUT,
BANKNIFTY-II,BANKNIFTY_26-NOV-2020_FUT,
NIFTY-I,NIFTY_24-SEP-2020_FUT,
Sample “quantity-mapping.csv”
- Column 1: Datafeed provider symbol
- Column 2: Quantity for the symbol
- Give a comma (,) at the end of each line
- See sample entries below, copy them & paste it into a csv file
BANKNIFTY-I,50,
BANKNIFTY-II,25,
NIFTY-I,75,
Trading in Multiple Accounts
There are two approaches to achieve trading into multiple accounts. You can go through them in Trading Strategies For Multiple Accounts.
Approach 1: One Strategy Instance Per Account
To trade in multiple accounts in AmiBroker, you need to run multiple charts or scanners. You need to have one chart/scanner per account.
Approach 2: One Strategy for All Accounts
A single strategy runs across multiple accounts. We have already provided multi-account trading sample AFLs in our library. See screenshots below:
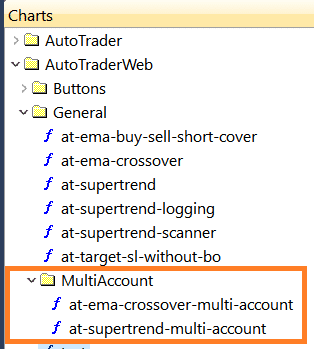

Place order on next Candle or Bar
This feature is useful to handle a specific scenario. Some times you will see that there are short lived signals. A short lived signal is one which appears on a bar or candle and disappears by the time the bar or candle is complete.
This problem can be avoided by placing the order on Next Bar or Candle. We have added this feature in our sample afls & you can set it to ON if you need to use it. See screenshot below:
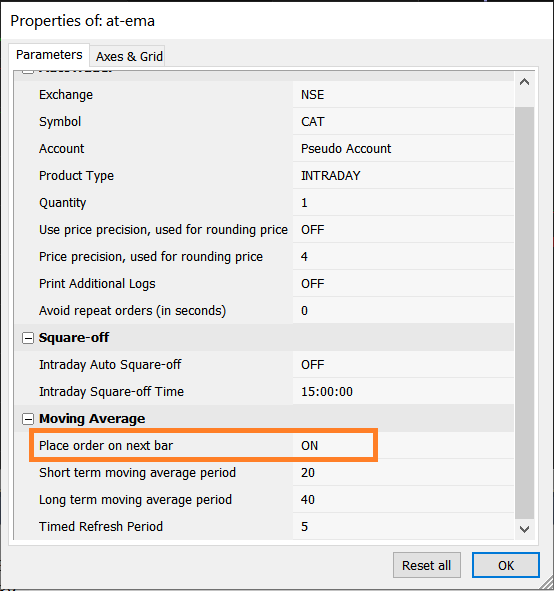
Debug
A simplified approach in debugging AFL code is to use _TRACE() function and observe TRACE logs. Let us first look at how to setup your AmiBroker to view logs.
- Increase _TRACE() output to max (5000 lines) (See screenshot below)
- Bring up the log window (AmiBroker Menu: Window -> Log)
- Go to the Trace tab & enable both Internal & External output (See screenshot below)
- Enable the switch to print additional logs in the parameters window (See screenshot below)
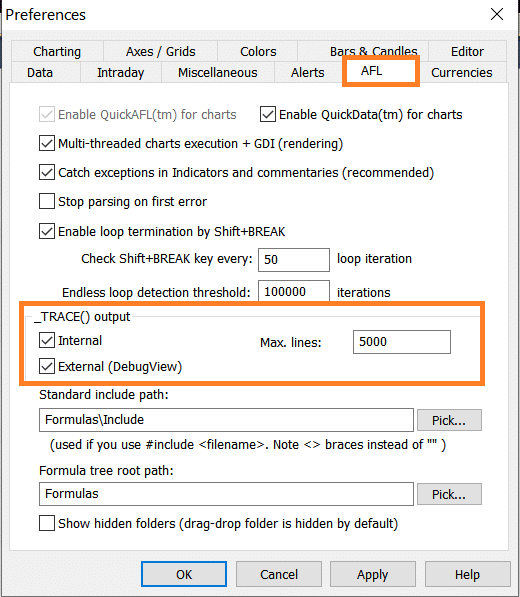
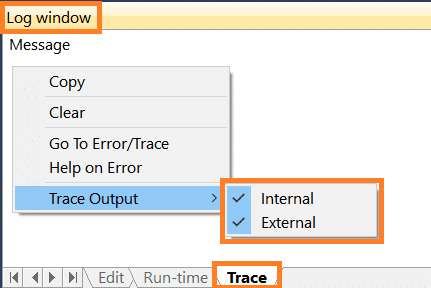
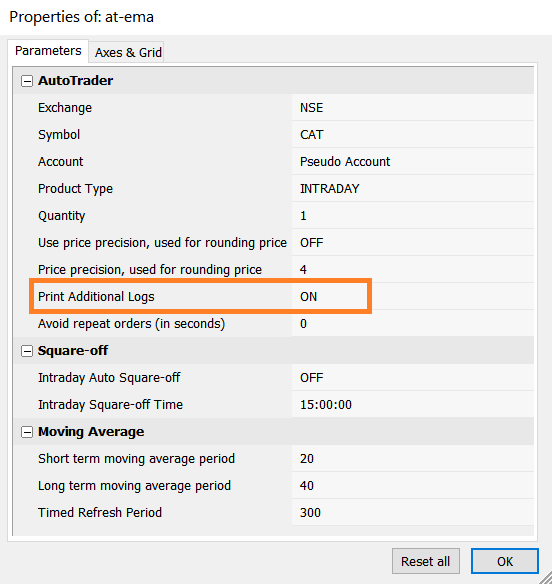
Logging
Logging is useful in understanding how your strategy is working during live market. It also helps in investigation of issues. It is pretty similar to _TRACE function mentioned above, but have more advantages. There is no limit on how long the file length can be & the logs are persisted even when AmiBroker is closed.
We provide open source AmiBroker utilities which include logging framework. You can read more about our open source library on it’s wiki.
You can configure file based logging in chart parameters. For more details, please see Logging library wiki.
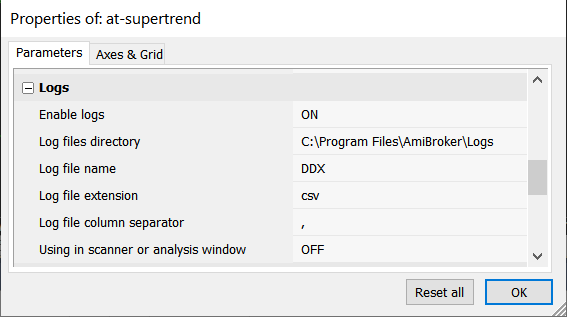
GitHub
If you are interested to look at the code of this library, it is available on GitHub.
Additional Information
You can find solution to all common issues in the knowledgebase.
Also be aware of this AmiBroker issue. Make sure you use RequestTimedRefresh() function as shown in the samples provided.
Compatibility with AmiBroker version 5.7 or below
If you are using version 5.7 or below, then we recommend upgrading the 5.8 or above. If that is not possible, then do the following.
- Just remove version check from this file. You will find the file in your AmiBroker’s Include folder.
- The fopen function used in the above file uses a flag called shared, which is only present from AmiBroker 5.8 onwards. You need to remove the shared flag.
- Note that you need to do above steps every time you re-install AmiBroker library. As reinstall replaces old files with latest ones from the repository.